Examples: Barcodes as a Service (Software as a Service, SaaS)
Thanks to over 300 edge servers distributed worldwide, barcode creation takes place in the cloud with low latency and maximum reliability. The software installation and maintenance is completely eliminated.
Here's an example of how to use it within your HTML page or web app:
SVG
This creates a barcode of the type Code 128 coding the text ActiveBarcode.
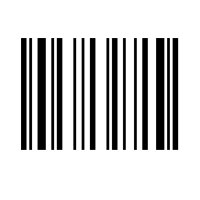
Highly accurate barcodes
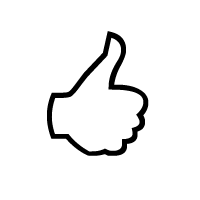
Server-side generation
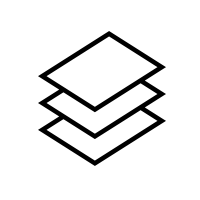
Automation
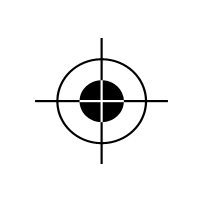
Platform independent
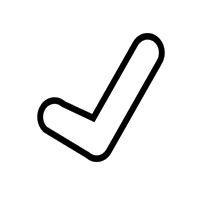
No installation & No maintenance
SVG
<img src="https://api.activebarcode.net/v2/svg?code=CODE128&text=ActiveBarcode&access=YOUR-KEY-HERE" />PNG
<img src="https://api.activebarcode.net/v2/png?code=CODE128&text=ActiveBarcode&access=YOUR-KEY-HERE" />
This creates a barcode of the type Code 128 coding the text ActiveBarcode.
- Parameters allow to create all barcodes types with properties as needed.
- You can try out and evaluate the REST API by omitting the API key. Without the access key, the barcode will be branded with a notice: "DEMO".
- The ActiveBarcode API creates SVG and PNG images to allow a wide range of applications.